Intro
I’ve been exploring unit testing and test coverage for JavaScript as I work on a new SharePoint app for SharePoint online in the Office 365 suite. The obvious research paths led me to Qunit.js and right after that, to Blanket.js.
QUnit let me set up unit tests and group them into modules. A module is just a simple way to organize related tests. (I’m not sure I’m using it as intended, but it’s working for me so far with the small set of tests I have thus far defined).
Blanket.js integrates with Qunit and it will show me the actual lines of JavaScript that were – and more importantly – were not actually executed in the course of running the tests. This is “coverage” – lines that executed are covered by the test while others are not.
Between setting up good test cases and viewing coverage, we can reduce the risk that our code has hidden defects. Good times.
Qunit
Assuming you have your Visual Studio project set up, start by downloading the JavaScript package from http://qunitjs.com. Add the JavaScript and corresponding CSS to your solution. Mine looks like this:
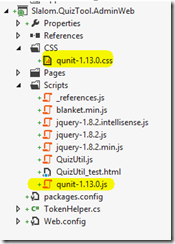
Figure 1
As you can see, I was using 1.13.0 at the time I wrote this blog post. Don’t forget to download and add the CSS file.
That out of the way, next step is to create some kind of test harness and reference the Qunit bits. I’m testing a bunch of functions in a script file called “QuizUtil.js” so I created an HTML page called “QuizUtil_test.html” as shown:
Figure 2
Here’s the code:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>QuizUtil test with Qunit</title>
<link rel="stylesheet" href="../CSS/qunit-1.13.0.css" />
<script type="text/javascript" src="QuizUtil.js" data-cover></script>
<script type="text/javascript" src="qunit-1.13.0.js"></script>
<script type="text/javascript" src="blanket.min.js"></script>
<script>
module("getIDFromLookup");
test("QuizUtil getIDFromLookupField", function () {
var goodValue = "1;#Paul Galvin";
equal(getIDFromLookupField(goodValue) + 1, 2), "ID of [" + goodValue + "] + 1 should be 2";
equal(getIDFromLookupField(undefined), undefined, "Undefined input argument should return undefined result.");
equal(getIDFromLookupField(""), undefined, "Empty input argument should return an undefined value.");
equal(getIDFromLookupField("gobbledigood3-thq;dkvn ada;skfja sdjfbvubvqrubqer0873407t534piutheqw;vn"), undefined,"Should always return a result convertible to an Integer");
equal(getIDFromLookupField("2;#some other person"), "2", "Checking [2;#some other person].");
equal(getIDFromLookupField("9834524;#long value"), "9834524", "Large value test.");
notEqual(getIDFromLookupField("5;#anyone", 6), 6, "Testing a notEqual (5 is not equal to 6 for this sample: [5;#anyone]");
});
module("htmlEscape");
test("QuizUtil htmlEscape()", function () {
equal(htmlEscape("<"), "<", "Escaping a less than operator ('<')");
equal(htmlEscape("<div class=\"someclass\">Some text</div>"), "<div class="someclass">Some text</div>", "More complex test string.");
});
module("getDateAsCaml");
test("QuizUtil getDateAsCaml()", function () {
equal(getDateAsCaml(new Date("12/31/2013")), "2013-12-31T:00:00:00", "Testing hard coded date: [12/31/2013]");
equal(getDateAsCaml(new Date("01/05/2014")), "2014-01-05T:00:00:00", "Testing hard coded date: [01/05/2014]");
equal(getDateAsCaml(new Date("01/31/2014")), "2014-01-31T:00:00:00", "Testing hard coded date: [01/31/2014]");
equal(getTodayAsCaml(), getDateAsCaml(new Date()), "getTodayAsCaml() should equal getDateAsCaml(new Date())");
equal(getDateAsCaml("nonsense value"), undefined, "Try to get the date of a nonsense value.");
equal(getDateAsCaml(undefined), undefined, "Try to get the date of the [undefined] date.");
});
module("getParameterByName");
test("QuizUtil getParameterByName (from the query string)", function () {
equal(getParameterByName(undefined), undefined, "Try to get undefined parameter should return undefined.");
equal(getParameterByName("does not exist"), undefined, "Try to get parameter value when we know the parameter does not exist.");
});
module("Cookies");
test("QuizUtil various cookie functions.", function () {
equal(setCookie("test", "1", -1), getCookieValue("test"), "Get a cookie I set should work.");
equal(setCookie("anycookie", "1", -1), true, "Setting a valid cooking should return 'true'.");
equal(setCookie("crazy cookie name !@#$%\"%\\^&*(()?/><.,", "1", -1), true, "Setting a bad cookie name should return 'false'.");
equal(setCookie(undefined, "1", -1), undefined, "Passing undefined as the cookie name.");
equal(getCookieValue("does not exist"), "", "Cookie does not exist test.");
});
</script>
</head>
<body>
<div id="qunit"></div>
<div id="qunit-fixture"></div>
</body>
</html>
There are several things happening here:
- Referencing my code (QuizUtil.js)
- Referencing Qunity.js
- Defining some modules (getIDFromLookup, Cookies, and others)
- Placing a <div> whose ID is “qunit”.
Then, I just pull up this page and you get something like this:
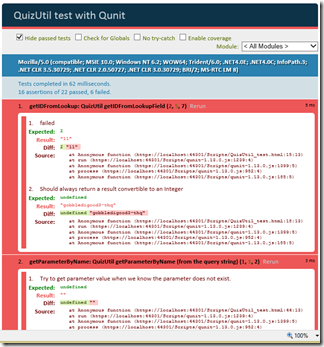
Figure 3
If you look across the top, you have a few options, two of which are interesting:
- Hide passed tests: Pretty obvious. Can help your eye just see the problem areas and not a lot of clutter.
- Module: (drop down): This will filter the tests down to just those groups of tests you want.
As for the tests themselves – a few comments:
- It goes without saying that you need to write your code such that it’s testable in the first place. Using the tool can help enforce that discipline. For instance, I had a function called “getTodayAsCaml()”. This isn’t very testable since it takes no input argument and to test it for equality, we’d need to constantly update the test code to reflect the current date. I refactored it by adding a data input parameter then passing the current date when I want today’s date in CAML format.
- The Qunit framework documents its own tests and it seems pretty robust. It can do simple things like testing for equality and also has support for ajax style calls (both “real” or mocked using your favorite mocker).
- Going through the process also forces you to think through edge cases – what happens with “undefined” or null is passed into a function. It makes it dead simple to test these scenarios out. Good stuff.
Coverage with Blanket.js
Blanket.js complements Qunit by tracking the actual lines of code that execute during the course of running your tests. It integrates right into Qunit so even though it’s a whole separate app, it plays nicely – it really looks like it’s one seamless app.
This is blanket.js in action:
Figure 4
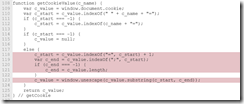
Figure 5
(You actually have to click on the “Enable coverage” checkbox at the top [see Figure 3] to enable this.)
The highlighted lines in Figure 5 have not been executed by any of my tests, so I need to devise a test that does cause them to execute if I want full coverage.
Get blanket.js working by following these steps:
- Download it from http://blanketjs.org/.
- Add it to your project
- Update your test harness page (QuizUtil_test.html in my case) as follows:
- Reference the code
- Decorate your <script> reference like this:
<script type="text/javascript" src="QuizUtil.js" data-cover></script>
Blanket.js picks up the “data-cover” attribute and does its magic. It hooks into Qunit, updates the UI to add the “Enable coverage” option and voila!
Summary (TL; DR)
Use Qunit to write your test cases.
- Download it
- Add it to your project
- Write a test harness page
- Create your tests
- Refactor some of your code to be testable
- Be creative! Think of crazy, impossible scenarios and test them anyway.
Use blanket.js to ensure coverage
- Make sure Qunit is working
- Download blanket.js and add it to your project
- Add it to your test harness page:
- Add a reference to blanket.js
- Add a “data-cover” attribute to your <script> tag
- Run your Qunit tests.
I never did any of this before and had some rudimentary stuff working in a handful of hours.
Happy testing!
</end>
Subscribe to my blog.
Follow me on Twitter at http://www.twitter.com/pagalvin